Prediction based on more than one features.
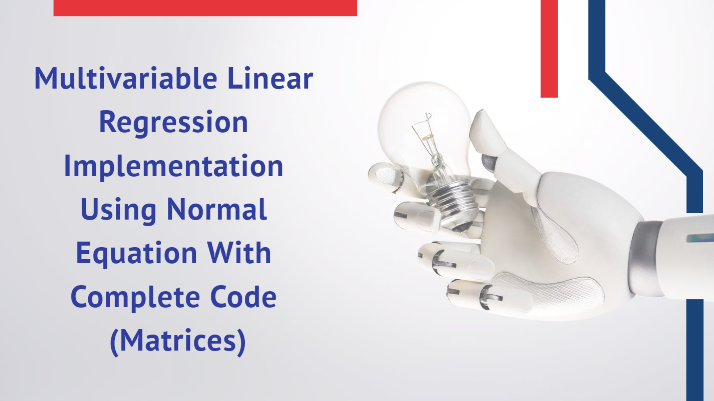
Hey guys, In my previous articles I show you how we can implement Simple Linear Regression using “Normal Equation” in python. Now what does it mean by Simple Linear Regression? It means that we are going to predict the value of output using only one feature from our dataset. Now it’s sometimes hard for us to predict the value from only one feature, right? So that’s why we need to use Multivariable Linear Regression. Now what happens in Multivariable Linear Regression? In MLR we predict the value of output from more than one input features. For example we can predict the price of house using it’s area, neighbourhood, number of bedrooms etc. So that’s when we can use Multivariable Linear Regression.
Normal Equation is as follow :
So let’s get started.
Here we are going to use a dataset that has columns named as age,credit-rating,children and loan. Our goal is to predict the value of loan based on the input features called age,credit-rating and children.
Hypothesis function for multivariate linear regression :
y = beta_0 + beta_1*x1 + beta_2*x2 + …..+ beta_n*xn
Let’s code!
(1) Import the required libraries :
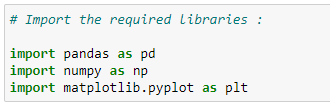
(2) Read the file :
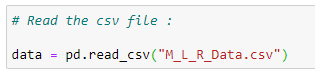
(3) Print the first five rows of dataset :
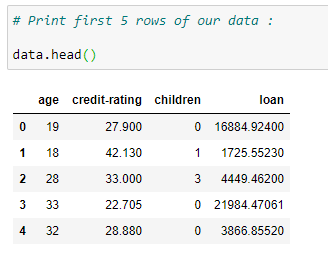
(4) Print column names :

(5) Read the column data into variables :
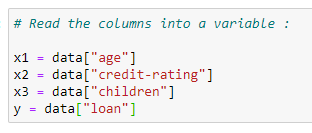
(6) Shape of our variables :
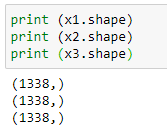
(7) Plot the data on scatter plot:
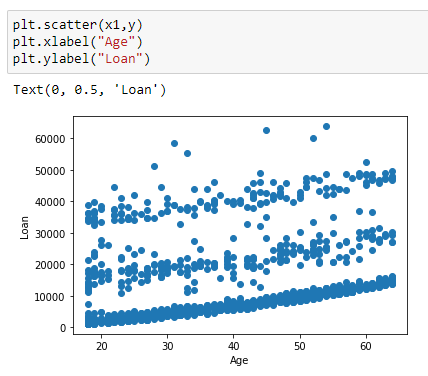
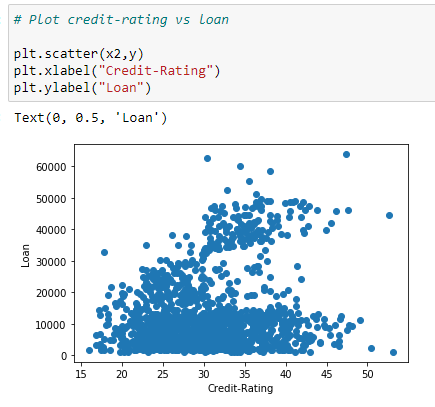
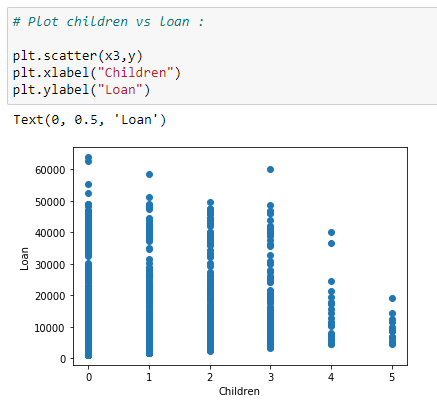
(8) Convert our variables datatype from series to array :
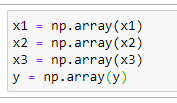
(9) Number of rows in our dataset :
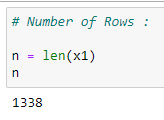
(10) Create a “ones” matrix :
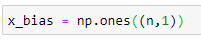
(11) Reshape our data so that we can perform operations like addition and multiplication with x_bias :
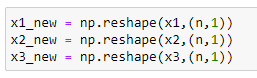
(12) Create a major matrix with all the columns like x_bias,x2,x2,x3 :
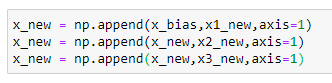
(13) Print the major matrix :
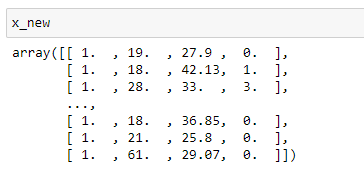
(14) Find transpose of a matrix :

(15) Perform multiplication :

(16) Find inverse :

(17) perform multiplication :
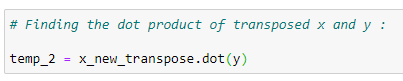
(18) Finding coefficients :
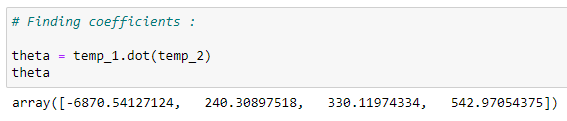
(19) print the coefficient values :
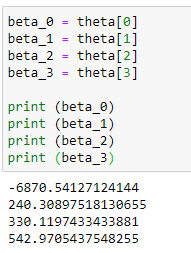
(20) Predict the values based on the calculated coefficient values :
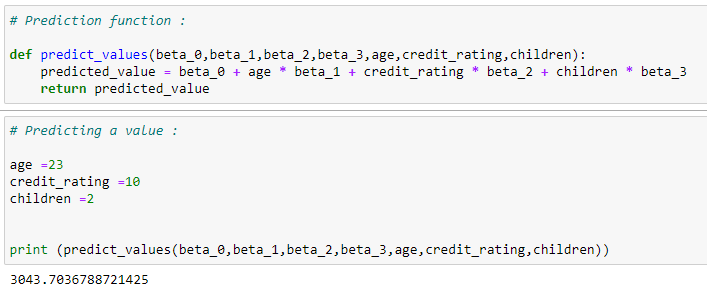
Okay. So this is how we can perform Multivariable Linear Regression using python.
Moving Forward,
All my articles are available on my blog :
patrickstar0110.blogspot.com
Watch detailed videos with explanations and derivation on my youtube channel :
(1) Simple Linear Regression Explained With It’s Derivation:
https://youtu.be/1M2-Fq6wl4M
(2)How to Calculate The Accuracy Of A Model In Linear Regression From Scratch :
https://youtu.be/bM3KmaghclY
(3) Simple Linear Regression Using Sklearn :
https://youtu.be/_VGjHF1X9oU
(4) Machine Learning Mathematic (Matrices) Explained :
https://youtu.be/1MASyeyAydw
(5) Complete Mathematical Derivation Of Normal Equation:
https://youtu.be/E7Q4UP6bNmc
(6) Simple Linear Regression Implementation Using Normal Equation:
https://youtu.be/wmmUJnmwQho
Read my other articles :
(1) Linear Regression From Scratch :
https://medium.com/@shuklapratik22/linear-regression-from-scratch-a3d21eff4e7c
(2) Linear Regression Through Brute Force :
https://medium.com/@shuklapratik22/linear-regression-line-through-brute-force-1bb6d8514712
(3) Linear Regression Complete Derivation:
https://medium.com/@shuklapratik22/linear-regression-complete-derivation-406f2859a09a
(4) Simple Linear Regression Implementation From Scratch:
https://medium.com/@shuklapratik22/simple-linear-regression-implementation-from-scratch-cb4a478c42bc
(5) Simple Linear Regression From Scratch :
https://medium.com/@shuklapratik22/simple-linear-regression-implementation-2fa88cd03e67
(6) Gradient Descent With it’s Mathematics :
https://medium.com/@shuklapratik22/what-is-gradient-descent-7eb078fd4cdd
(7) Linear Regression With Gradient Descent From Scratch :
https://medium.com/@shuklapratik22/linear-regression-with-gradient-descent-from-scratch-d03dfa90d04c
(8) Error Calculation Techniques For Linear Regression :
https://medium.com/@shuklapratik22/error-calculation-techniques-for-linear-regression-ae436b682f90
(9) Introduction to Matrices For Machine Learning :
https://medium.com/@shuklapratik22/introduction-to-matrices-for-machine-learning-8aa0ce456975
(10) Understanding Mathematics Behind Normal Equation In Linear Regression (Complete Derivation)
https://medium.com/@shuklapratik22/understanding-mathematics-behind-normal-equation-in-linear-regression-aa20dc5a0961
(11)Implementation Of Simple Linear Regression Using Normal Equation(Matrices)
https://medium.com/@shuklapratik22/implementation-of-simple-linear-regression-using-normal-equation-matrices-f9021c3590da
Thanks for posting this info. I just want to let you know that I just check out your site and I find it very interesting and informative. I can't wait to read lots of your posts. machine learning interview questions
ReplyDelete